Initially when REST was introduced there was always challenge to validate your request against prerequisite requirement. This was available in SOAP web services as XSD schema validation but it was not available in REST webservice. Architect and developer had to face the challeng to implement some kind of schema to validate their request.
YAML based RAML (Restful API modeling Language) was introduced in 2013. RAML gives flexibility to define schema to validation request/response. This breakthrough helps Architect and developer to define schema for REST API to validate request/response.
RAML schema validation can be defined in two formats.
1) XSD Based
2) Json Based.
Schema validation can be defined in two ways inside RAML
1) Inline schema definition
2) XSD or json schema definition file.
Schema definition can be defined in schema tag within RAML file.
“!Include” tag uses to include schema file for file based schema definition within RAML.
Json based schema definition
/car:
post:
description: Getting car info from Car Application
body:
application/json:
schema: !include schemas/cars-schema-request.json
XSD based schema definition
responses:
200:
body:
application/xml:
schema: !include schemas/vanrish-car-response.xsd
Inline definition of schema
/car:
post:
description: Getting car info from Car Application
body:
application/json:
schema: |
{
"type": "object",
"$schema": "http://json-schema.org/draft-03/schema",
"required": true,
"properties": {
"vin": {
"type": "string",
"required": true
},
"model": {
"type": "string",
"required": true
},
"make": {
"type": "string",
"required": true
}
}
}
Here are few tips to use json based schema validation.
1) If request/response is object based then in schema it is defined as type:Object .
"$schema": "http://json-schema.org/draft-03/schema",
"type": "object"
2) If request/response is list based then it is defined as
"$schema": "http://json-schema.org/draft-03/schema",
"type": "array",
"items": {
3) If request/response is list and it contain object, it is defined as
"items": {
"type": "object",
"properties": {
"vin": {
"type": "string"
}
4) Type of field for Object can be defined as string,integer, or boolean
"vin": {
"type": "integer"
}
5) Field can be restricted for known value with defining enum
"isCdl": {
"description": "State",
"type": "string",
"enum": [ "true", "false" ]
}
6) Field can be made mandatory by introducing required field
"name": {
"type": "string",
"required": true
}
7) Any field can be validated against regular expression by defining regex code
"deviceName": {
"type": "string",
"pattern": "^/dev/[^/]+(/[^/]+)*$"
}
8) Maximum and minimum field length can be validated with defining maxLength and minLength field
"id": {
"description": "A three-letter id",
"type": "string",
"maxLength": 3,
"minLength": 3
}
9) Reuse of validation by importing validated schema from different file system by defining like that
"credentials": { "$ref": "app-credential.json#/definitions/credential" }
10) anyOf, allOf, oneOf, not- these four keywords intend to bring logical processing primitives to Schema validation
{
"anyOf": [
{ "type": [ "string", "boolean" ] },
{ "schema1": "#/definitions/nfs" },
{ "schema2": "#/definitions/pfs" }
]
}
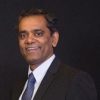
Rajnish Kumar is CTO of Vanrish Technology with Over 25 years experience in different industries and technology. He is very passionate about innovation and latest technology like APIs, IOT (Internet Of Things), Artificial Intelligence (AI) ecosystem and Cybersecurity. He present his idea in different platforms and help customer to their digital transformation journey.
Thank you Rajnish. very helpful!
Thanks Pascal !!
Hi Rajneesh Sir,
Need your help in one of my project.
I am new in mule and i want to validate request body of the api.
below is the example:
Raml Info:
/getnameValidate:
post:
responses:
200:
headers:
body:
application/json:
example: |
{
“success”: true,
“message”: “name validated successfully”
}
API calling using Postman:
URL: https://localhost:8081/api/getnameValidate
Body:
{
“name”: “vishal”
}
validation should be like ..name should not be null or empty .please help me or suggest me how can i do that.
Thanks & Regards,
Vishal